by Team InDeepData
   
1751
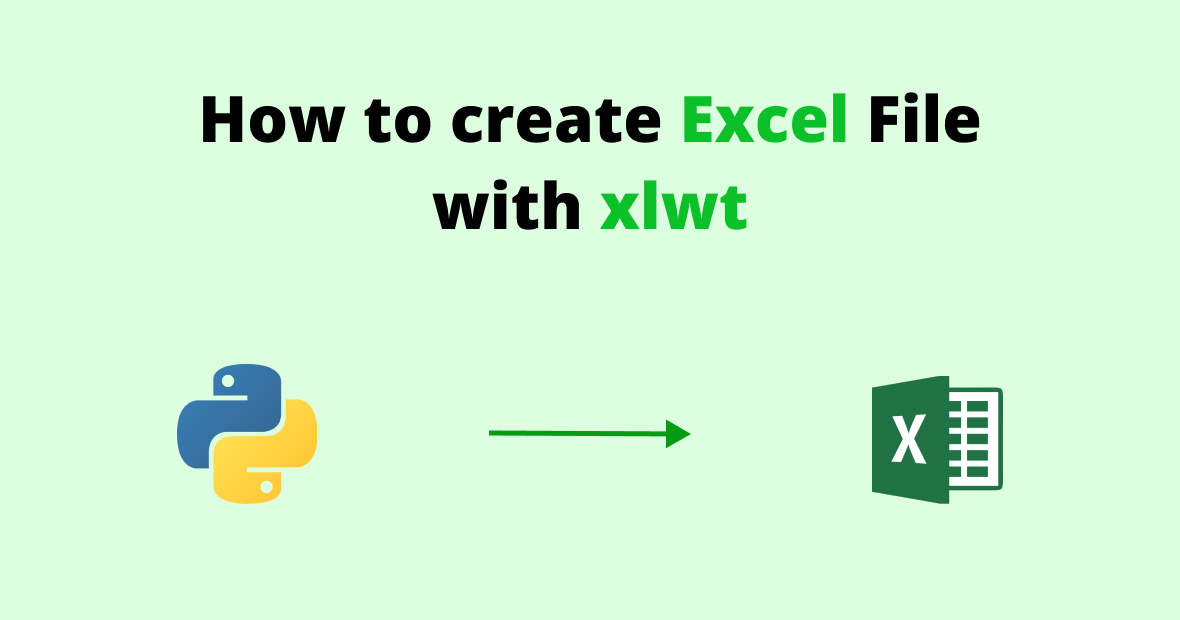
Python's XLWT
library allows you to write Excel files. In addition to text and numbers, formulas can also be entered into multiple worksheets. Other features include styling text, managing column size, and more.
Table of Contents
How to install xlwt librabry
Install xlwt with the below command.
pip install xlwt
In order to test our code after installation, we need to create a dummy data set.
data = [
{
"name":"Testuser1",
"age":20,
"country":"India"
},
{
"name":"Testuser2",
"age":20,
"country":"Canada"
},
{
"name":"Testuser2",
"age":29,
"country":"USA"
},
]
Now we have the data to create excel file.
How to create Excel with Python using xlwt
Please follow the below given code
# Import workbook to write data from xlwt
from xlwt import Workbook
# Create an object of the workbook
excel = Workbook()
# Add sheet in workbook
sheet = excel.add_sheet("Test Data")
for index, value in enumerate(data):
sheet.write(index, 0, value["name"])
sheet.write(index, 1, value["age"])
sheet.write(index, 2, value["country"])
# Now save the excel
save_location = "path/to/your/working/directory/result.xls"
excel.save(save_location)
Thanks for visiting InDeepData