by Team InDeepData
8484
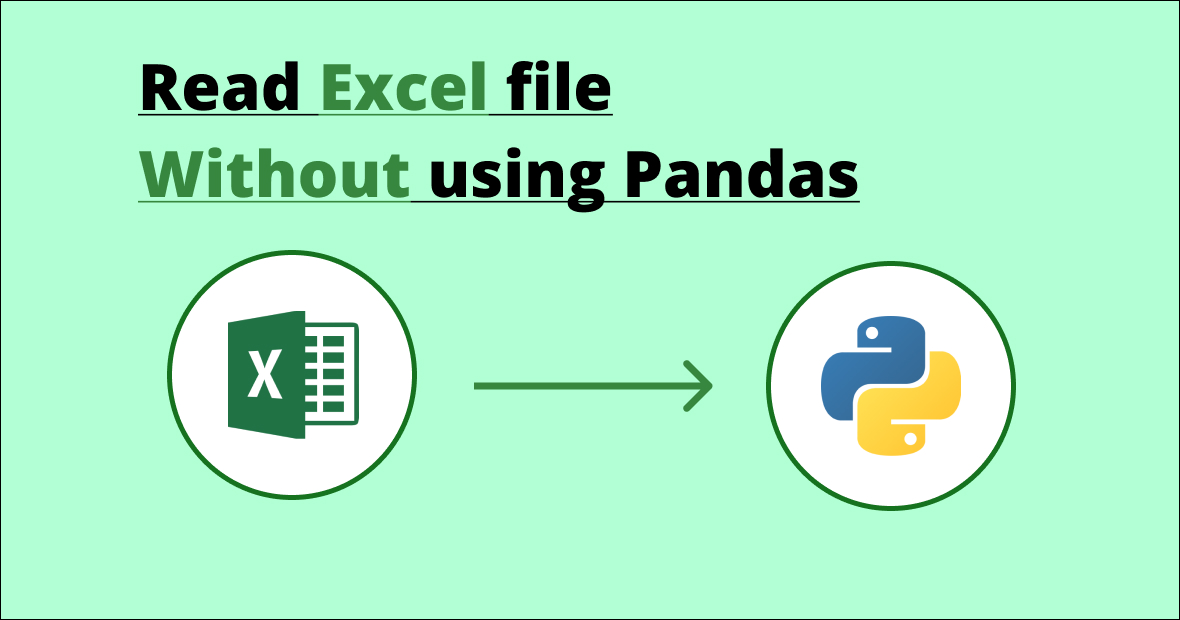
Reading of an Excel files with extensions(.xls, .xlsx) can be done without using Pandas in Python programming. Instead of using Pandas we can use other alternative libraries like xlrd, openpyxl
.
In this article we will see how we can read Excel file in Python by using xlrd
library.
Related Article: How to create Excel file with xlwt
Table of Contents
Install xlrd
First of all we have to install xlrd in our local environment. And xlrd can be installed using pip (Python package manager).
Use the given command to install it.
pip install xlrd
Read Excel with xlrd
Method open_workbook
will opens up the given Excel file so that we can read that file.
import xlrd
# Open the Excel file
workbook = xlrd.open_workbook('test.xls') # Replace 'your_excel_file.xls' with your file path
# Select the sheet you want to read (by index)
sheet = workbook.sheet_by_index(0)
# Iterate through rows and columns to access data
for row in range(sheet.nrows):
for col in range(sheet.ncols):
cell_value = sheet.cell_value(row, col)
print(cell_value, end='\t')
print() # Move to the next row
# Close the Excel file (not required for reading, but good practice)
workbook.release_resources()
To read a perticular sheet in the Whole Excel file we can select sheets by sheet_by_index
method.
Note: nrows and ncols will measure and tell how many rows and coulmns we have in a sheet.
Hopefully you are able to read Excel files with xlrd.