by Team InDeepData
2884
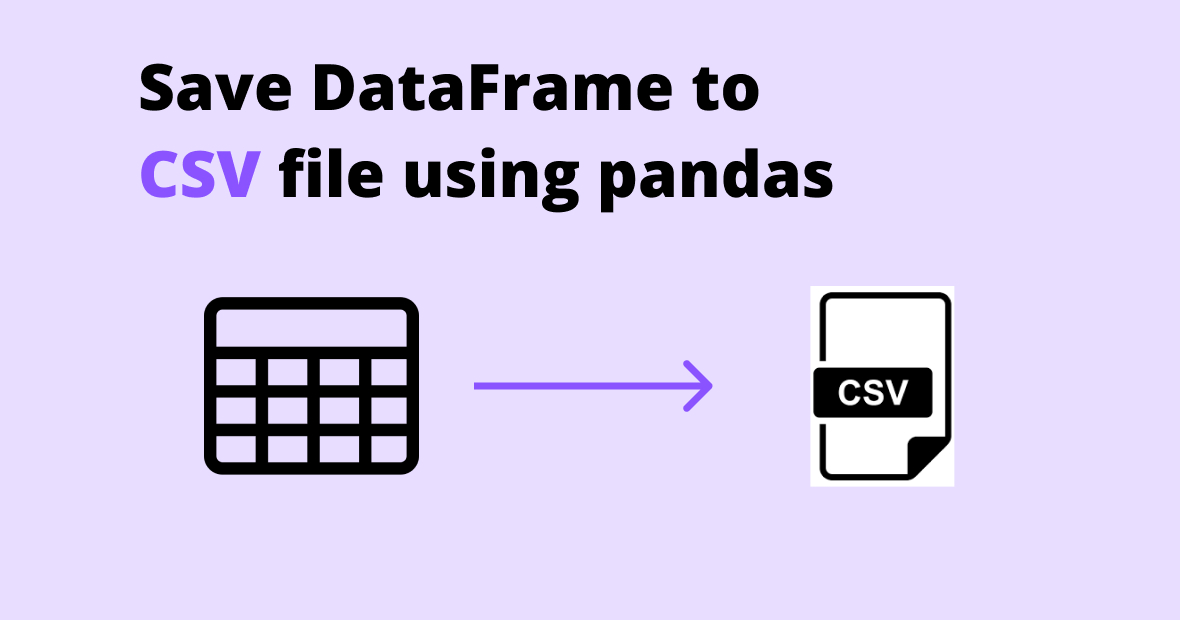
It is well known that Pandas is a popular library for manipulating and analyzing data. Additionally, it is widely used in Python and is open-source.
Most of the time, we find that the data is enormous when we begin analyzing it. Once we analyzed the data, we discovered some columns were not necessary or sometimes we needed to preprocess it. It could be anything. Once the data has been refined, we want to save it locally so we can use it in the future without having to repeat the entire process.
Pandas have a method .to_csv()
for storing data into CSV files which are exported and saved locally.
The following code demonstrates how it works.
import pandas as pd
# Create a simple data frame
data = {
'Name': ['John', 'Mary', 'James', 'Linda', 'Robert'],
'Age': [28, 23, 31, 19, 36],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix']
}
df = pd.DataFrame(data)
# Write the dataframe object into a CSV file
df.to_csv("/path/to/your/csvfile.csv", index=False, sep=',', encoding='utf-8')
print("File Created Successfully")
Parameters used in .to_csv
functions.
- The first parameter specifies the name of the CSV file (in my case, it's "csvfile.csv"). You would need to specify the full path if the file is not in the same directory as your script.
- index=False means that we will not write row names (index). If you want to include the index, you can set index=True or remove this parameter because its default value is True.
- sep=',' defines the delimiter to use. In this case, we use a comma.
- encoding='utf-8' specifies the encoding to be used for the file. This is optional, and if not specified, it will default to 'utf-8'.
This script will create the csvfile.csv
file in the same directory after it is run. You can create the file in another directory by replacing csvfile.csv
with the full path.
An example would be:
import pandas as pd
# Create a simple data frame
data = {
'Name': ['John', 'Mary', 'James', 'Linda', 'Robert'],
'Age': [28, 23, 31, 19, 36],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix']
}
df = pd.DataFrame(data)
# Write the data frame object into a CSV file
df.to_csv("/path/to/your/csvfile.csv", index=False, sep=',', encoding='utf-8')
print("File Created Successfully")
Make sure you replace /path/to/your/csvfile.csv
with your actual CSV file path.
Hopefully 🙌 this blog helps you.